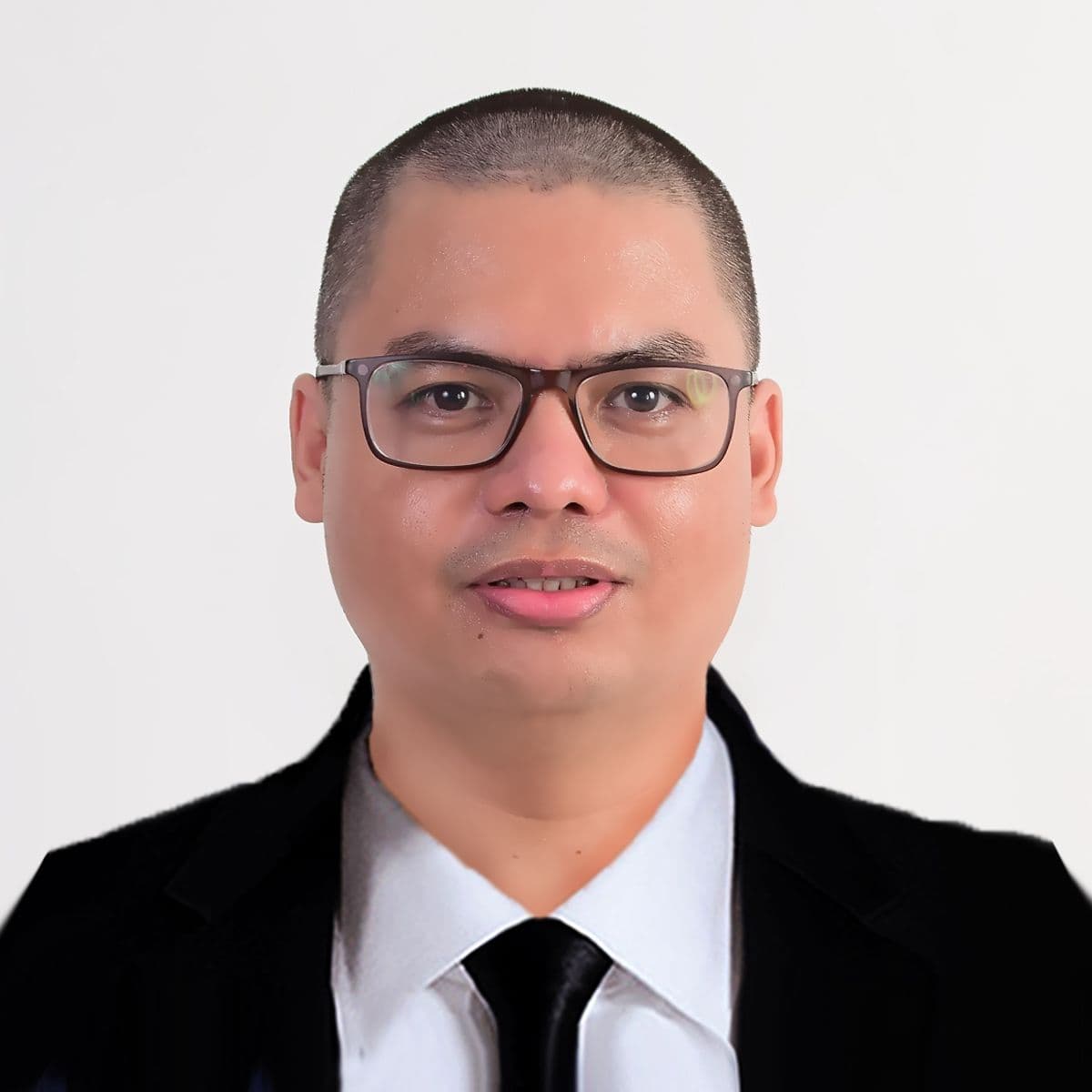
Test
March 9, 2023

Lucky Me
This is the description of blog #1.
Blog
---
title: How to use Reduce in JavaScript
tags:
- javascript
- fundamentals
- arrays
-reduce
date: 2020-05-09
excerpt: The reduce function of an array is one of the most versatile functions in JavaScript. With it you can accomplish a lot of the functions from array and math objects.
---
The [reduce](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/reduce) function of an `Array` is one of the most versatile functions in JavaScript. With it you can accomplish a lot of the functions from the [Array](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array) and [Math](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Math) objects. It's job is to start with an array, and reduce those elements into some other type of data... which sounds vague, but you could use it to convert from an array to an object, an array to another array, an array to a number or an array to a boolean. Understanding how it works opens up a world of possibilities.
<YouTube id="NiLUGy1Mh4U" />
A few of the Math/Array functions we will cover in this article:
- [Math.min()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Math/min)
- [Math.max()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Math/max)
- [Array.length](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/length)
- [Array.prototype.map()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/map)
- [Array.prototype.find()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/find)
- [Array.prototype.every()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/every)
- [Array.prototype.some()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/some)
- [Array.prototype.flat()](https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Array/flat)
## Let's Recreate Reduce Before we see all of reduce's flexibility, let's understand how it works! Reduce's job is to iterate over each element of an array, calling a function (callback), passing both the current element, and what is called an "accumulator" value. The callback's job is to take the accumulator and the array element, and return the new version of that accmulator.
<Image src="/images/reduce.jpg" width={1703} height={980} />
This may mean taking an accumulator that is a number and returning its value + 1, or taking an accumulator which is an array and returning that array with an additional element on the end. It's up to you, depending what type of data you would like to _reduce_ your array to.
We also need an _initial value_, which will be the first value our accumulator takes before the callback is ever called.
```js
function reduce(array, callback, initial) {
// start our accumulator off as the initial value
let acc = initial;
// iterate over each element in the array
for (let i = 0; i < array.length; i++) {
// pass the accumulator and current element to callback function
// override the accumulator with the callback's response
acc = callback(acc, array[i], i); }
// return the final accumulator value
return acc;
}
result = reduce([1, 2, 3], (acc, num) => acc + num, 0);
```